Don't use early returns to handle simple cases
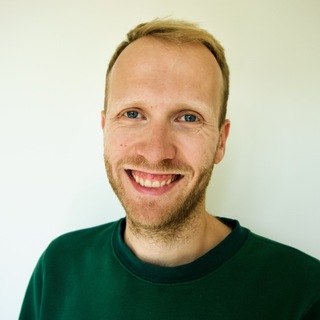
- Name
- Dennis Paagman
- @djfpaagman
Early returns are a wonderful thing. They make your code readable and cases where the code can be cut short are clear.
They can also be overused in cases where a case
statement would be preferred. Take this example:
def country_code(country)
return "NL" if country == "Netherlands"
"DE"
end
Rewritten using a case
statement it reads much nicer:
def country_code(country)
case country
when "Netherlands" then "NL"
else "DE"
end
This has a few other benefits as well:
- Adding new cases is obvious, just add another
when
statement. - It exposes another issue: should the German case really be handled in the
else
? It’s more clear to add an explicitwhen
for it as well. - Preprocessing of the variable is easier, it only requires a change in one line of code.
It could evolve into something like this:
def country_code(country)
case country.chomp
when "Netherlands" then "NL"
when "Germany" then "DE"
when "France" then "FR"
end
Save the early return for more complex methods where non-trivial code paths can be skipped and cut short.