Using empty if statements for clarity
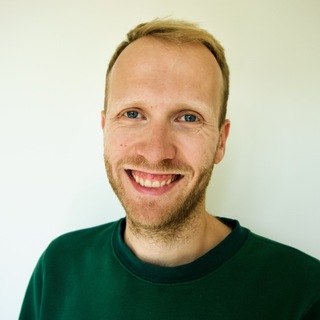
- Name
- Dennis Paagman
- @djfpaagman
Recently I wrote a piece of code that needed to do nothing. It was covering an edge case where we had to rescue an exception and ignore it in a certain situation.
The code I ended up with:
rescue IOError => e
if e.message == "closed stream"
# the hard shutdown! will error out with an IOError exception, so we just swallow that.
else
raise e
end
end
Only after writing it I realized that itβs not the most straight forward way to write code like this. More common would be an early return, for example:
rescue IOError => e
# the hard shutdown! will error out with an IOError exception, so we just swallow that.
return if e.message == "closed stream"
raise e
end
Or in a one liner:
rescue IOError => e
# the hard shutdown! will error out with an IOError exception, so we just swallow that.
raise e unless e.message == "closed stream"
end
But I like the empty if statement. It clearly shows weβre doing nothing in a very specific case, and what is done elsewhile. Itβs easy to read, as it uses the most simple and common pattern in code, the if .. else statement. It signals the purpose of the code, swallowing that one edge case in the most simple way.
Clear code is good code.